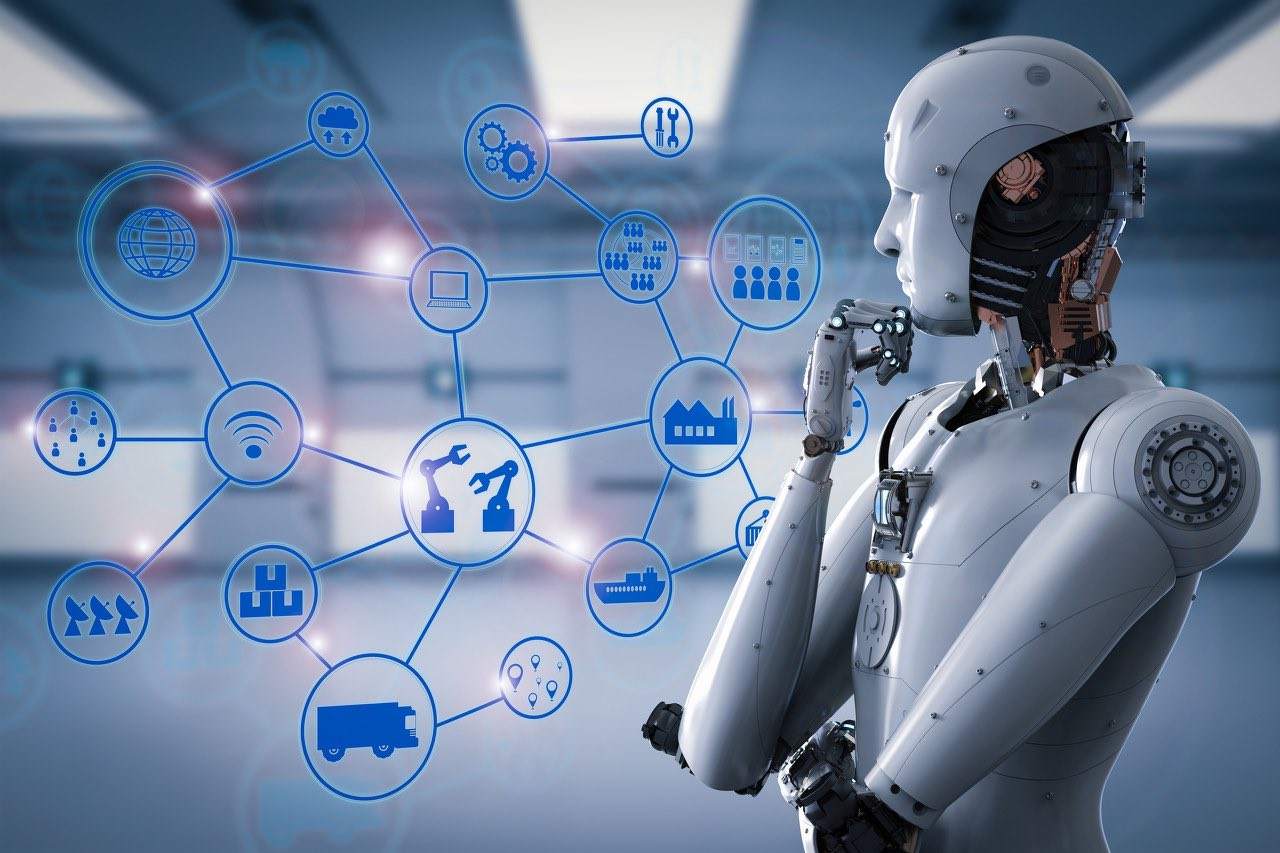
Promise和Async/Await有什么区别?
1、Async/Await是什么?
我们先从字面上理解,async顾名思义是异步的意思,函数前面加个async表示该函数是异步函数,而await是async wait的缩写,就是等待异步执行完的意思。
function asyncCode() {
return Promise.resolve("hello async")
}
上面一个简单函数,如果我们用正常promise.then是这样执行的:
asyncCode().then(res=>{
console.log(res)
// 打印出来"hello async"
})
如果用async/await的话,我们可以这样写:
async function asyncCode(){
return "hello async"
}
const n = asyncCode();
console.log(n)
//1. 返回一个promise对象
const n1 = asyncCode();
n1.then(res=>{
console.log(res)
})
//2. 打印hello async
const n2 = await asyncCode();
console.log(n2)
//3. 打印hello async
上面两段函数的含义完全相同,带async函数其实返回的就是一个promise.resolve函数,如果函数没有return,那就是resolve了一个undefined
2、Async/Await有什么作用?
既然async函数调用的就是promise函数,那么有什么作用呢?
Async/Await的优势在于处理多重then链,让代码看起来更优雅
/**
* 传入参数 n,表示这个函数执行的时间(毫秒)
* 执行的结果是 n + 200,这个值将用于下一步骤
*/
function takeLongTime(n) {
return new Promise(resolve => {
setTimeout(() => resolve(n + 200), n);
});
}
function step1(n) {
console.log(`step1 with ${n}`);
return takeLongTime(n);
}
function step2(n) {
console.log(`step2 with ${n}`);
return takeLongTime(n);
}
function step3(n) {
console.log(`step3 with ${n}`);
return takeLongTime(n);
}
现在用 Promise 方式来实现这三个步骤的处理
function doIt() {
console.time("doIt");
const time1 = 300;
step1(time1)
.then(time2 => step2(time2))
.then(time3 => step3(time3))
.then(result => {
console.log(`result is ${result}`);
console.timeEnd("doIt");
});
}
doIt();
// c:\var\test>node --harmony_async_await .
// step1 with 300
// step2 with 500
// step3 with 700
// result is 900
// doIt: 1507.251ms
输出结果 result
是 step3()
的参数 700 + 200
= 900
。doIt()
顺序执行了三个步骤,一共用了 300 + 500 + 700 = 1500
毫秒,和 console.time()/console.timeEnd()
计算的结果一致。
如果用 async/await 来实现呢,会是这样
async function doIt() {
console.time("doIt");
const time1 = 300;
const time2 = await step1(time1);
const time3 = await step2(time2);
const result = await step3(time3);
console.log(`result is ${result}`);
console.timeEnd("doIt");
}
doIt();
结果和之前的 Promise 实现是一样的,但是这个代码看起来是不是清晰得多,几乎跟同步代码一样,详细推荐阮一峰老师讲解